This is a slight modification of K9MGM‘s solution to get your AmbientWeather 2902 data to APRS-IS via API.
Note: If you don’t want to host the service yourself, you can sign up for free at AmbientCWOP.com, just enter your AmbientWeather API Key and callsign, it will collect the data for you!
The original solution involved using Amazon’s AWS/Lambda scripting but I found that a little complicated, and I like to keep my stuff “in-house” so to speak.
This is the Python script, slightly modified to run on Linux, in my case I run it on a Synology DiskStation using Task Scheduler to run it every 10 minutes (below the script).
You’ll need to create an API and Application Key from your AmbientWeather control panel.
import urllib, json, time, http.client
from urllib.request import urlopen
# REPLACE THESE VALUES ########
mac = 'YOUR AMBIENT WEATHER MAC'
apiKey = 'YOUR API KEY'
applicationKey = 'THE APPLICATION KEY'
# Your call and substation ID go here, e.g. KN4DVB-1
callAndSSID = 'CALLSIGN'
# Your APRS passcode - https://apps.magicbug.co.uk/passcode/
aprsPasscode = str('12345')
# latitude must be exactly 7 chars - include leading zeros
latitude = '3300.12N'
# longitude must be exactly 9 chars - include leading zero(s)
longitude = '12233.80W'
destination = 'APRS'
#uncomment (remove the # from) the following line if you are a real, trained NWS SKYWARN watcher
#destination = 'SKY'
#########################
url = 'https://api.ambientweather.net/v1/devices/' + mac + '?apiKey=' + apiKey + '&applicationKey=' + applicationKey + '&endDate=&limit=1'
json_url = urlopen(url)
data = json.loads(json_url.read())
diff = time.time() - (data[0]["dateutc"]/1000)
responsebody = ""
if (diff < 800):
postbody = 'user ' + callAndSSID +' pass ' + aprsPasscode +'\n'
dhmtime = str(data[0]["date"][8:10]) + str(data[0]["date"][11:13]) + str(data[0]["date"][14:16]) + "z"
postbody += callAndSSID + '>' + destination + ',WIDE1-1:@' + dhmtime + latitude + '/' + longitude + '_'
# wind direction
postbody += 'c'
postbody += (str('000') + str(data[0]["winddir"]))[-3:]
# wind speed
postbody += 's'
postbody += (str('000') + str(round(float(data[0]["windspeedmph"]))))[-3:]
# wind gust
postbody += 'g'
postbody += (str('000') + str(round(float(data[0]["windgustmph"]))))[-3:]
# temp F
postbody += 't'
tempf = int(data[0]["tempf"])
if (tempf < 0):
postbody += "-" + (str("00") + str(tempf))[-2:]
else:
postbody += (str("000")+str(tempf))[-3:]
# rain in last hour
postbody += 'r'
postbody += (str('000') + str(int(data[0]["hourlyrainin"]*100)))[-3:]
# rain in last day
postbody += 'P'
postbody += (str('000') + str(int(data[0]["dailyrainin"]*100)))[-3:]
#humidity
postbody += "h"
postbody += (str('00') + str(data[0]["humidity"]))[-2:]
#pressure
postbody += "b"
postbody += (str('00000') + str(int(10*33.864*data[0]["baromrelin"])))[-5:]
# Comment line, put whatever you like
postbody += "This is my wonderful script"
posturl = "http://rotate.aprs2.net:8080/"
conn = http.client.HTTPConnection('rotate.aprs2.net',8080)
headers = {'Content-Type':'application/octet-stream','Accept-Type':'text/plain'}
conn.request('POST', '/', postbody, headers)
responsebody = str(conn.getresponse().read())
# Uncomment these lines if you want to see the output
# print(responsebody)
# print(postbody)
Lat/Long
You can use this conversion utility to convert your Lat/Long to minutes/seconds. The format is MMM.DD
Corrections Made
url string was missing the application key variable &applicationKey=’ + applicationKey
DiskStation Setup
With Python installed on your DiskStation, and the script saved in your home folder, open Control Panel, Task Scheduler (under Services)
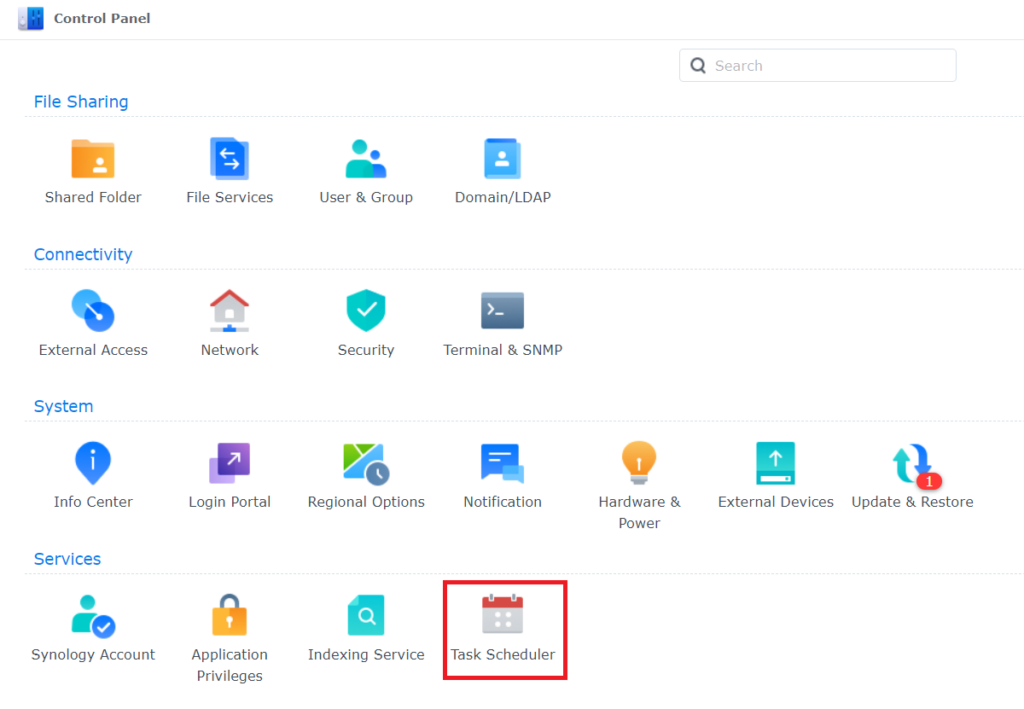
Click Create > Scheduled Task
General Tab
Task: APRS Push
User: (your admin name)
Schedule Tab
Run Daily
Time / First Run Time: 00:00
Frequency: Every 10 minutes
Last Run Time: 23:50
Task Settings Tab
Under Run Command:
/bin/python /var/services/homes/(your admin name)/aprs.py
Click OK to save.
That’s it! You can highlight your new scheduled task entry and click Run to kick it off right away. You should see if moments later in the aprs.fi website.